C String
- A group of an array which consists of characters, ASCII numbers and, special characters is called a character array. When an array consists of integer value it is named as integer array and, when an array consists of characters, ASCII numbers, and special characters then it is named a character array. The character array is also called a string.
- The string consists of the null character at its end which is represented by the ‘\0’ symbol. Each character array is terminated by the ‘\0’ symbol.
- There is a difference between the ‘\0’ and 0. The ASCII value of 0 is 48 and the ASCII value of the ‘\0’ is 0.
- It is very important to terminate the string by ‘\0’ because by using the ‘\0’ we can detect where the string ends.
Initialization of string::
- char name[]={'P','R','O','G','R','A','M','\0'};
we can also initialize as,
- char name[]= “ PROGRAM”;
Here the declaration of null character at the end is not necessary because C inserts the null character at the end automatically.
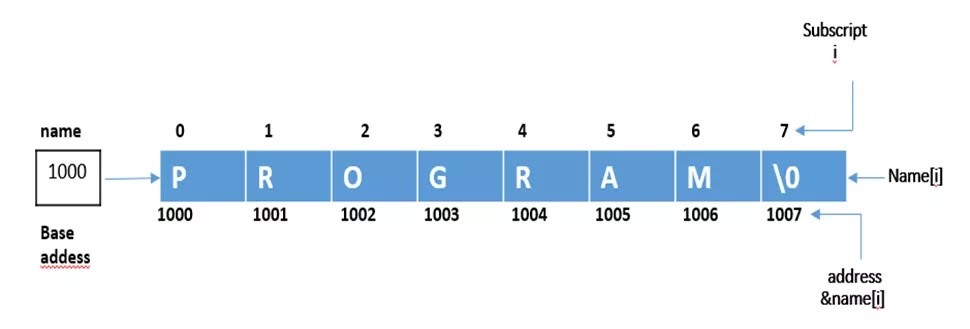
Representation of string
Lets perform some operation on given
array::
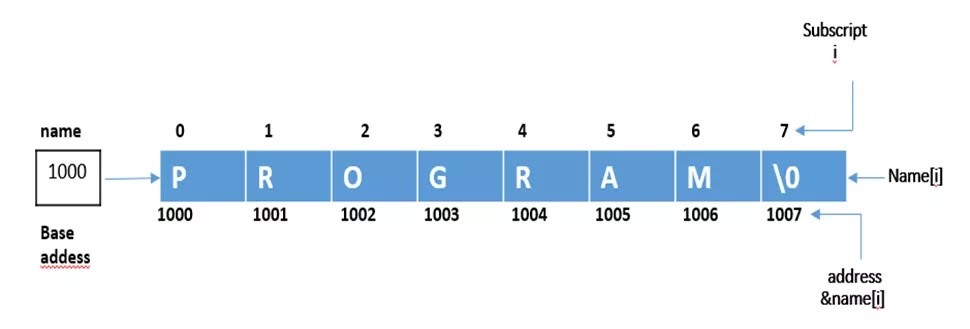
- printf(“%s”, name);
Output:: PROGRAM
- printf(“%c”, name[3]);
Output:: G
- printf(“%d”, name[3]);
Output:: 103
// prints ASCII value of G
- printf(“%u”, name);
Output:: 1000
// prints base address
- printf(“%u”, &name[3]);
Output:: 1003
// prints address of block we G is inserted
- printf(“%s”, &name[3]);
Output:: GRAM
// prints word from G
- printf(“%s”,&name[4]);
Output:: RAM
Initialization of one-dimensional string
There are three ways to initialize the string
1. #include<stidio.h>
int main()
{
char a[ ]= “program”;
int i;
for(i=0;i<=6;i++)
{
printf(“%c”, a[i]);
}
return 0;
}
Output:: program
Explanation: In the first program, we have just used simple for loop and incremented it till 6, as there are seven characters in the string, hence the loop will run from 0 to 6. In this method, we have to know the length of the string.
2. #include<stidio.h>
int main()
{
char a[ ]= “program”;
int i;
for(i=0;a[i]!=’\0’;i++)
{
printf(“%c”, a[i]);
}
return 0;
}
Output:: program
Explanation: In the second method we have used for loop and the for loop works until the null character ‘\0’ comes. As we know that by default at the end of every string there is the null character. Generally, this way of programming is used in the string, in this method, we don’t need to know the length of the string.
3. #include<stidio.h>
int main()
{
char a[ ]= “program”;
char *ptr;
int i;
ptr=a;
for(i=0;*ptr!=’\0’;ptr++)
{
printf(“%c”, *ptr);
}
return 0;
}
Output:: program
Explanation: In the third method we have printed the string with the help of using a pointer. Pointer stores the address of another variable.
![]() |
representation-pointer-c |
We can print the whole string without using any loop
#include<stdio.h>int main()
{
char
a[10]=”program”;
printf(“%s “, a);
return 0;
}
Output:: program
// %s is a format specifier for printing out a string
By using the scanf we cannot print the sentence of characters such as “god is great”
This sentence cannot be printed using scanf because it considers the spaces as a null character so, instead of
Scanf() we use gets() which can accept a hole sentence and can count the spaces and instead of Printf() we use puts()
As shown in the below program.
#include<stdio.h>
int main()
{
char a[20];
printf(“enter the sentence:\n”);
gets(a); // receiving input
puts(“print”);
puts(a); //printing output
return 0;
}
Output:: enter the sentence:
god is great
god is great
Also, refer::
Two-dimensional string |
string functions in c |
1 Comments
I like ur explaination and representation ...it helps to understand this topic
ReplyDeletePost a Comment